Today, we are going to see how we can use pointers and arrays in C++. We hope now you have some basic understanding of pointers. If don’t, we would highly recommend going through the last tutorial and coming back here. Now let us discuss the basics of pointers quickly then we will start with today’s agenda.
Pointers
A pointer is a variable that stores an object’s memory address. In both C and C++, pointers are used widely for three major purposes: allocating new objects on the heap, passing functions to other functions, and so on. to cycle through the elements of arrays or other data structures.
Syntax: datatype* pointerVariableName = &variable;
Let’s consider a basic example of pointer pointing to first element of an array.
#include <iostream>
using namespace std;
int main() {
int* ptrArray;
int arr[] = { 1, 2, 3, 4, 5 };
ptrArray = arr;
return 0;
}
In this example you can see we have declared a pointer of integer type and then declared an array, finally we assign an array to the pointer. But wait, we have discussed in the last tutorial pointer stores address, how can we assign array to pointer. This is because by default when we assign an array to a pointer, the address of the first element is stored in the pointer (ptrArray = &arr[0]). Yes, the only first element, and using this pointer we can iterate on the whole array. That means, ptrArray + 1 will be equivalent to &arr[1] and so on. Let’s now try to see the address of all elements using that one pointer.
Code
#include <iostream>
using namespace std;
int main() {
int* ptrArray;
int size = 5;
int arr[] = { 1, 2, 3, 4, 5 };
ptrArray = arr;
for (int iter = 1; iter <= size - 1; iter++) {
cout << "Array element " << arr[iter] << " has address " << &arr[iter] << " And ptrArray + " << iter <<
" is " << ptrArray + iter << "\n";
}
return 0;
}
Output

We have printed addresses of the remaining array elements and (pointer + iteration index) and they both have the same address. Now, let’s print array elements using that pointer. Remember the concept of dereferencing the pointer, we will utilize it.
#include <iostream>
using namespace std;
int main() {
int* ptrArray;
int size = 5;
int arr[] = { 1, 2, 3, 4, 5 };
ptrArray = arr;
cout << "\n======Displaying Array======\n";
for (int iter = 0; iter < size; iter++) {
cout << "Array at pos " << iter << " is: " << *(arr + iter) << "\n";
}
cout << "=================================";
return 0;
}
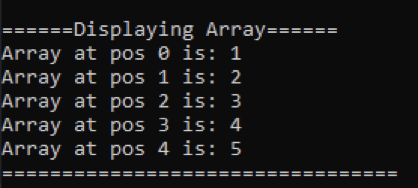
Alternatively, we could have used arr[iter] for accessing elements, both work fine.
Let’s do an example where we used the array name as a pointer, ask the user to populate an array and finally, we will display the array.
#include <iostream>
using namespace std;
#define size 5 // constant that store size of an array
// method declarations
void populate(int*); // this method used to populate an array
void display(int*); // this method used to display an array
int main() {
int arr[size];
//calling populate method to populate an array
populate(arr);
//displaying an array by calling display method
display(arr);
return 0;
}
// Method definitions
void populate(int* arr) {
for (int iter = 0; iter < size; iter++) {
cout << "Enter element for index " << iter<<": ";
cin >> *(arr + iter);
}
}
void display(int* arr) {
for (int iter = 0; iter < size; iter++) {
cout << "Element at index " << iter << " is: "<< *(arr+iter) << "\n";
}
}
Output
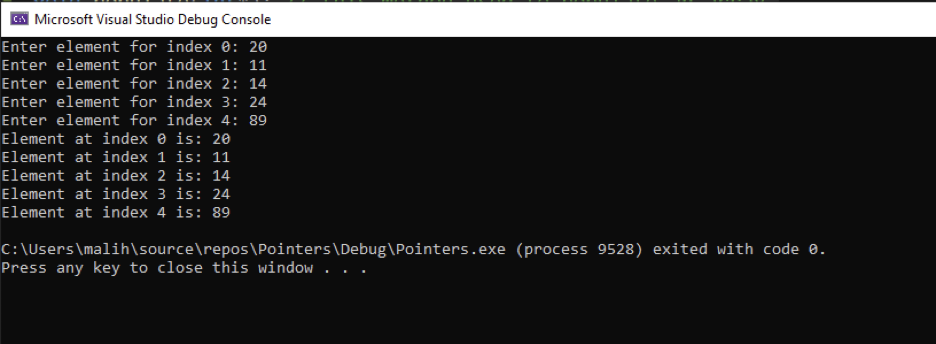
You can see we have passed the array to methods that receive it as a pointer. The rest of the concepts are the same, as we have discussed earlier.
Conclusion
We hope you enjoyed this tutorial and that you learned something. Thanks for reading and stay tuned with us for more such amazing tutorials.