Welcome back to the tutorial for the detection of faces using OpenCV with c++. If you don’t know what this library is, we would highly recommend going through the previous tutorial and the setup project. Let me explain a little about Open CV before diving into it.
OpenCV
OpenCV (Open-Source Computer Vision Library) is a free software library for computer vision and machine learning. It was created to provide a common infrastructure for computer vision applications and to help commercial goods incorporate machine perception more quickly. Because it is a BSD-licensed product, it is simple for businesses to use and alter the code.
Writing the code
Let us create a new file named source.cpp and paste the following code inside it.
#include<iostream>
#include<stdlib.h>
#include<string.h>
#include<opencv2\highgui\highgui.hpp>
#include<opencv2\imgproc\imgproc.hpp>
#include<opencv2\objdetect\objdetect.hpp>
using namespace std;
using namespace cv;
/*
* This Method will detect face in image.
* Params:
* 1- img of type Matrix.
* 2- output Path of Image
* Returns:
* Void
*
*/
void detect_faces_(Mat, string);
CascadeClassifier detector;
int main() {
if (!detector.load("C:\\Users\\malih\\Downloads\\opencv\\sources\\data\\haarcascades\\haarcascade_frontalface_default.xml")) {
cout << "\n Failed to Load Classifier.";
exit(0);
}
string image_path = "C:\\Users\\malih\\Desktop\\download.jpg";
Mat image = imread(image_path, IMREAD_UNCHANGED);
// Now we will check if image is failed to load we will throw an error
if (image.empty()) {
cout << "\n failed to load "<< image;
}
string output_path = "C:\\Users\\malih\\Desktop\\output.jpg";
detect_faces_(image, output_path);
return 0;
}
void detect_faces_(Mat img, string output_path) {
vector<Rect> facesFound;
detector.detectMultiScale(img, facesFound);
for (int i = 0; i < facesFound.size(); i++) {
Point point1(facesFound[i].x, facesFound[i].y);
Point point2((facesFound[i].x + facesFound[i].height), (facesFound[i].y + facesFound[i].width));
rectangle(img, point1, point2, Scalar(0, 255, 0), 2, 8, 0);
}
imwrite(output_path, img);
cout << "Successfully detected face...";
}
And yes, don’t forget to correct the path of haarcascade. Run the code with the cpp compiler of your choice.
We will get the following output.
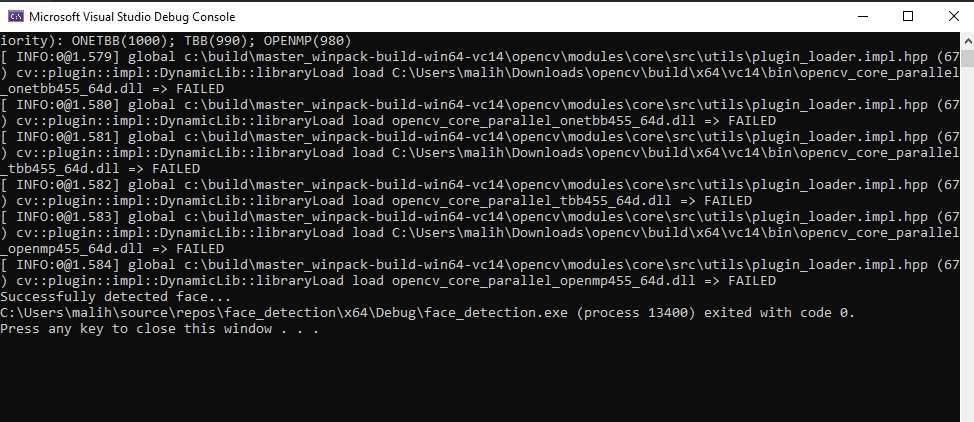
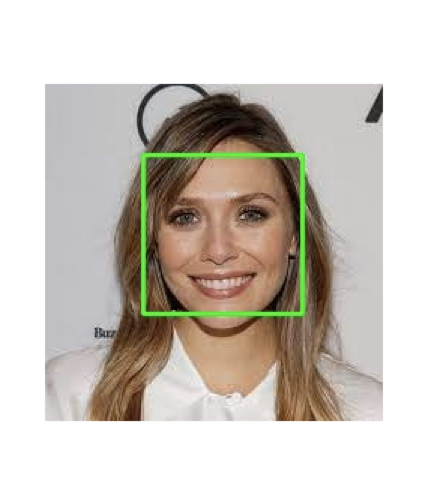
our classifier is working fine. One thing we want to clarify is that when you are specifying project setup don’t forget to choose architecture type to x64. It’s very important else your code won’t work.
Working Of the code
The library has in-built classifiers that have the required algorithms for detection of a face inside the image. In the above code, we initialize the CascadeClassifier named detector which is for detecting the face in an image. The function detector.detectMultiScale takes the input of an image and in return, we get the coordinates of a rectangle that surrounds the face. In the case of multiple faces, we get a list of rectangle coordinates. In our code, we create a rectangle vector for the output and draw it using green points.
Note: It may possible you may encounter a ***.dll not found error, In that case, open project properties, under debug go to environments, and then edit and paste the following line.
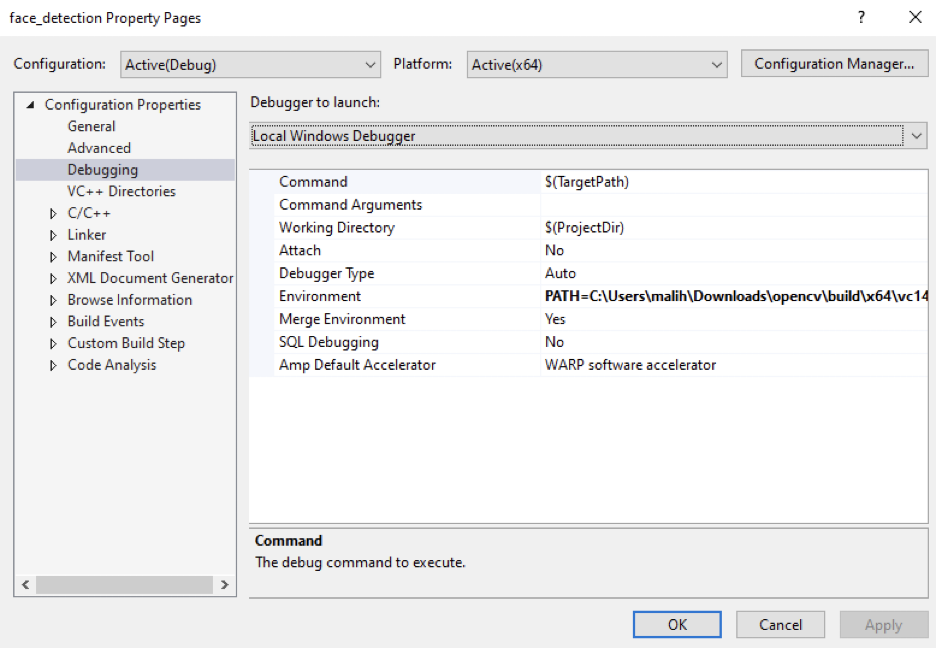
PATH=opencv/builds/x64/vc14/bin and then apply. Restart your compiler and the error will be fixed.
Conclusion
In this article, we saw a simple face detection program using OpenCV with c++. We would like to recommend practicing code yourself to get hands-on practice on it. Read more about OpenCV at https://opencv.org/releases/