Hey folks! Today we are going to see delete operator for deallocating dynamic memory in C++, I strongly recommend going through part I of this tutorial to have important and basic concepts of Dynamic Memory.
Delete Operator
In most circumstances, a program needs dynamically allocated memory only for some specific duration or use case. Therefore, when unused, we free it to accommodate more memory allocation requests. This is the purpose of the delete operator
Syntax
Delete pointer;
Delete[] pointer;
The first statement frees memory for a single element allocated with new, while the second statement frees memory for arrays of items allocated with new and a size in brackets ([]). Either a pointer to a memory block previously allocated with new or a null pointer must be given as an input to remove (in the case of a null pointer, delete produces no effect).
Let’s now see all this in action. If you followed the previous tutorial then just copy-paste the below code in your main.cpp file, otherwise create an empty directory open it with the editor of your choice, and then create an empty file namely main.cpp, and put the following code inside it.
#include <iostream>
using namespace std;
int main() {
//Taking array size from user
int size;
cout << "Enter size of an array: ";
cin >> size;
//declaring and allocating dynamic memory with notthrow object
int* studentsMarks;
studentsMarks = new (nothrow) int[size];
if (studentsMarks == nullptr) {
cout << "Failed to allocate Memory";
}
else {
//populating array
for (int i = 0; i < size; i++) {
cout << "Enter Marks for student " << i + 1 << ": ";
int marks;
cin >> marks;
studentsMarks[i] = marks;
/*
* We could have used, *(studentMarks + i) = marks instead of
* studentsMarks[i] = marks;
*/
}
//displaying array
for (int i = 0; i < size; i++) {
cout << "Marks for student " << i + 1 << " are: " << studentsMarks[i] << endl;
}
//now we will simply deallocate memory by using delete[]
cout << "Deallocationg memory...\n";
delete[] studentsMarks;
cout << "Deallocated successfully...\n";
}
return 0;
}
Output
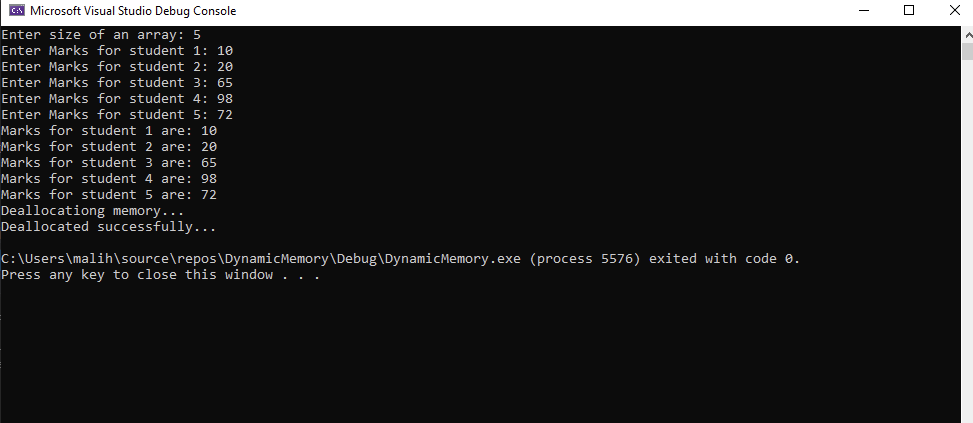
Interestingly, Let’s try to access array after deallocating memory. For this, I am just putting this code after deallocation of memory.
cout << "Trying to access memory after deallocation...\n";
for (int i = 0; i < size; i++) {
cout << "Marks for student " << i + 1 << " are: " << studentsMarks[i] << endl;
}
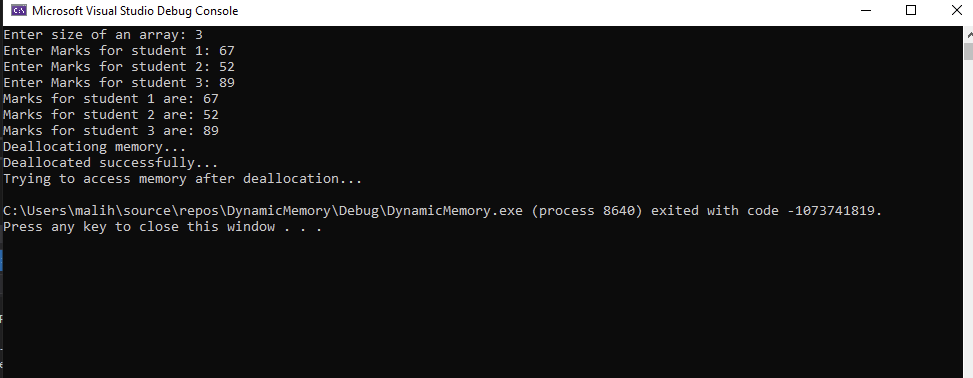
And you can see our program exits with some weird code, which means our program fails to exit successfully.
And let’s try another thing, when the program asks for the size of the array, we will give something very big, and let’s see whether we have a memory allocation error or not.
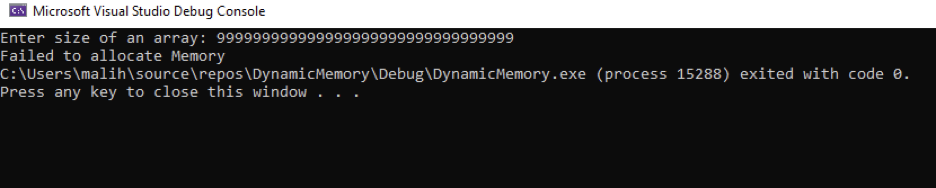
Finally, let’s try to resize array which we can’t when dealing with static arrays. Put the following code in main.cpp file.
#include <iostream>
using namespace std;
// ======== Method Declarations ======
void Resize(int*, int &);
int main() {
//Taking array size from user
int size;
cout << "Enter size of an array: ";
cin >> size;
//declaring and allocating dynamic memory with notthrow object
int* studentsMarks;
studentsMarks = new (nothrow) int[size];
if (studentsMarks == nullptr) {
cout << "Failed to allocate Memory";
}
else {
//populating array
for (int i = 0; i < size; i++) {
cout << "Enter Marks for student " << i + 1 << ": ";
int marks;
cin >> marks;
studentsMarks[i] = marks;
/*
* We could have used, *(studentMarks + i) = marks instead of
* studentsMarks[i] = marks;
*/
}
//displaying array
for (int i = 0; i < size; i++) {
cout << "Marks for student " << i + 1 << " are: " << studentsMarks[i] << endl;
}
//now we will resize our array
cout << "Resizing Array ....\n";
Resize(studentsMarks, size);
cout << "Successfuly resized ...\n";
//lets now check whether size is updated or now
cout << "New Array Size is: " << size << endl;
//displaying array
for (int i = 0; i < size; i++) {
cout << "Marks for student " << i + 1 << " are: " << studentsMarks[i] << endl;
}
cout << "Deallocating memory now ...\n";
delete[] studentsMarks;
cout << "Successfully deallocated...\n";
}
return 0;
}
// ========= Method Definitions ============
void Resize(int* array, int& size) {
//we will double size of old array
int newSize = size * 2;
int* newArray = new int[newSize];
//now we will use memcpy to copy old array into new one
memcpy(newArray, array, size * sizeof(int));
//updating size
size = newSize;
//updating old array to new
array = newArray;
//deleting array
delete[] newArray;
}
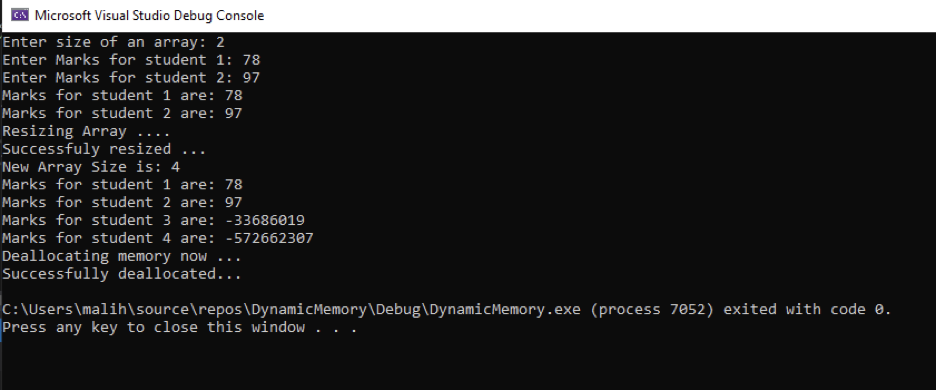
Conclusion
Now you can see the advantage of nothrow, and dynamic arrays. So, that’s it for today’s tutorial. I hope you enjoyed it. Stay tuned for more such amazing tutorials. You can read more about dynamic memory here.