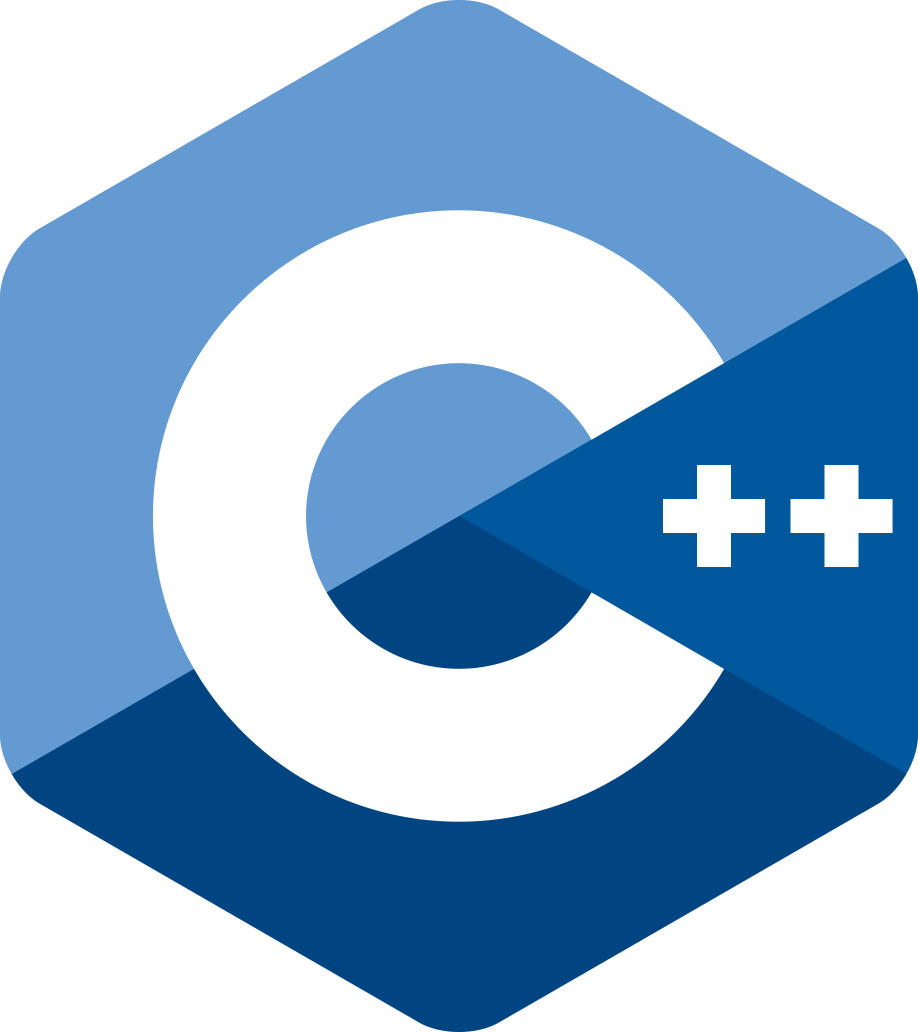
Introduction
What is Object Oriented Programming(OOP)?
Object-oriented programming is a programming paradigm that organizes code around data(objects), rather than functions and logic. An object can be defined as a data field that has unique attributes and behavior.
For example, let’s take an object and call it Car
:
object Car;
This Car
instance can have many attributes:
object Car {
property manufacturer
property model
property releasedYear
property horsePower
}
In this case, the Car
is a class and its attributes(properties) are manufacturer
,model
, releasedYear
and horsePower
.
Furthermore, an object is an instance of a class.
//Tesla is now an object of the Car class
Tesla = new Car({manufacturer:"Tesla", model:"CyberTruck", releasedYear:"2019", horsePower:"1800"});
Using C++
Classes and Objects
To create a class, we can use the class
keyword like so:
class Car {
public:
string manufacturer;
string model;
int weight;
}
Here, we are telling C++ to create a class along with a handful of properties.
To use it in action, write the following code:
#include <iostream>;
using namespace std;
int main() {
Car Tesla; //create a new Car instance.
Tesla.manufacturer= "Tesla"; //assign the manufacturer property
Tesla.model= "CyberTruck";
cout <<Tesla.manufacturer<< endl; //log out their values
cout <<Tesla.model <<endl;
return 0;
}

Using functions
Just like properties, we can even append functions within our class definition:
class Car {
public:
//further code..
void sayHello() {
//when executed, log out the manufacturer property.
cout << "Hello! I am " << manufacturer << endl;
}
}
int main() {
//further code..
Tesla.sayHello(); //execute the sayHello method.
This will be the output of the code:

Inheritance
Inheritance is a part of the OOP paradigm. It means that another class will inherit the properties of another class.
For example, look at the following code:
class Vehicle {
numberOfTyres;
size;
weight;
}
We can create another class called Car
that can inherit from Vehicle’s properties:
class Car : Vehicle { //inherits from Vehicle class
//other than Vehicle's properties, it will also have the following properties;
horsePower;
seats;
}
//create a Car instance.
Car Tesla;
Tesla.numberOfTyres = 4; //since Car is a child class of Vehicle, we can use this property.
Tesla.horsePower =1500;
To use inheritance with C++, write the following code:
#include <iostream>;
using namespace std;
class Vehicle {
public:
int numberOfTyres;
int size;
int weight;
};
class Car: public Vehicle { //tells C++ to
public:
string manufacturer;
string model;
};
int main() {
Car Tesla;
Tesla.numberOfTyres = 4; //use numberOfTyres property from Vehicle class
Tesla.size= 400;
cout<<Tesla.numberOfTyres<<endl;
return 0;
}
In this piece of code, we created a Car
class which is a child class of Vehicle
. Later on, we built a Car
instance and assigned their properties.
This will be the result of the code:

Object Slicing
According to Stack Overflow, object slicing is a crucial concept in the programming space.
“Slicing” is where you assign an object of a derived class to an instance of a base class, thereby losing part of the information – some of it is “sliced” away.
As an example, see this code:
class A {
int foo;
};
class B : public A { //classs B inherits from A
int bar; //other than 'foo', the B class has another property called 'bar'.
};
After this step, if you were to write this:
B b;
b.bar = 100;
A a = b;
This means that the information in b
about the bar
property is lost in a
.
This problem is called slicing, which results in program instability and bugs.
Conclusion
In this article, you learned the fundamentals of OOP in the C++ programming language. OOP is a crucial concept not only in C++, but also in other languages as well.
Thank you so much for reading! Happy coding!